Table Of Content
- Design Patterns Index
- Structural Software Design Patterns in Java
- Step 3: Implement Concrete Elements:
- Software Design Pattern in other Programming Languages
- Strategy is a behavioral design pattern that lets you define a family of algorithms, put each of them into a separate…
- Use Cases of the Visitor Design Pattern

In this guide, we'll explore some of the most common design patterns in Java, complete with examples and code snippets. The Visitor Design Pattern is a behavioral design pattern that enables the separation of algorithms or operations from the objects they operate on. It allows you to define new operations on a collection of objects without modifying their classes directly. A design pattern is a well-established and documented solution to a common software problem. You can think of them as best practices used by knowledgeable and experienced software developers. In the quarter century since Java was first introduced, software developers have faced numerous general problems during software development.
Design Patterns Index
In this code, we’ve created a State interface and two concrete states that implement the State interface. We also have a Context class that uses the State interface to change its behavior based on its current state. The Decorator pattern is useful for adding and removing responsibilities from objects at runtime. It provides a flexible alternative to subclassing for extending functionality. However, it can result in many small objects that can be hard to track and debug.
Structural Software Design Patterns in Java
The iterator pattern is one of the behavioral patterns and is used to provide a standard way to traverse through a group of objects. The iterator pattern is widely used in Java Collection Framework where the iterator interface provides methods for traversing through a Collection. This pattern is also used to provide different kinds of iterators based on our requirements. The iterator pattern hides the actual implementation of traversal through the Collection and client programs use iterator methods.
Top 7 Courses to Become a Software Architect or Solution Architect - hackernoon.com
Top 7 Courses to Become a Software Architect or Solution Architect.
Posted: Tue, 28 Jan 2020 08:00:00 GMT [source]
Step 3: Implement Concrete Elements:
ML Design Pattern #5: Repeatable sampling by Lak Lakshmanan - Towards Data Science
ML Design Pattern #5: Repeatable sampling by Lak Lakshmanan.
Posted: Thu, 07 Nov 2019 08:00:00 GMT [source]
Other concepts, like the SOLID principles and clean code practices, are also crucial for writing efficient, maintainable code. It also encapsulates state-specific behavior and divides the behavior of a class. However, the State pattern can be overkill if a state machine has only a few states or rarely changes.
Being familiar with them and knowing them by heart can help you increase the speed and quality of your development. Design Patterns are simply sets of standardized practices used in the software development industry. They represent solutions, provided by the community, to common problems faced in every-day tasks regarding software development. The memento design pattern is used when we want to save the state of an object so that we can restore it later on.
Strategy is a behavioral design pattern that lets you define a family of algorithms, put each of them into a separate…
A design pattern can be considered as block that can be placed in your design document, and you have to implement the design pattern with your application. It's available inPDF/ePUB/MOBI formats and includes thearchive with code examples inJava, C#, C++, PHP, Python, Ruby,Go, Swift, & TypeScript. Let’s consider a simplified scenario where you have a shopping cart containing different types of products, and you want to apply different types of discounts to the products in the cart. The Visitor Pattern can help you achieve this by allowing you to define discount calculation logic in separate visitor classes. You have to implement the “initialize” step or “read data” step for each and leave other steps to do their operations with default behavior. Then, let another class named “DiscountFactory” create different kinds of Discounts based on the data.
Structural Design Patterns
We will cover the creational, structural, and behavioral design patterns, and provide practical examples of each pattern in action. By the end of this guide, you will have a solid understanding of how to implement design patterns in Java and how they can help you to write better code. Design patterns are reusable solutions to common problems that arise during software design. They provide a standardized way to approach these problems, making it easier for developers to create efficient and maintainable code.
Use Cases of the Visitor Design Pattern
The implementation of the singleton pattern has always been a controversial topic among developers. A design pattern is a generic repeatable solution to a frequently occurring problem in software design that is used in software engineering. It is a description or model for problem-solving that may be applied in a variety of contexts.
Provides a simplified interface to a library, a framework, or any other complex set of classes. Lets you ensure that a class has only one instance, while providing a global access point to this instance. Javatpoint provides tutorials with examples, code snippets, and practical insights, making it suitable for both beginners and experienced developers. Through this article, I have listed what a Factory Design Pattern is and how to implement a Factory. I hope to receive contributions from everyone so that the programming community can continue to develop, and especially so that future articles can be better. AccountType consists of fixed values that have been predefined in the banking industry domain; therefore, it is a constant, and we use Enum for this data type to ensure integrity.
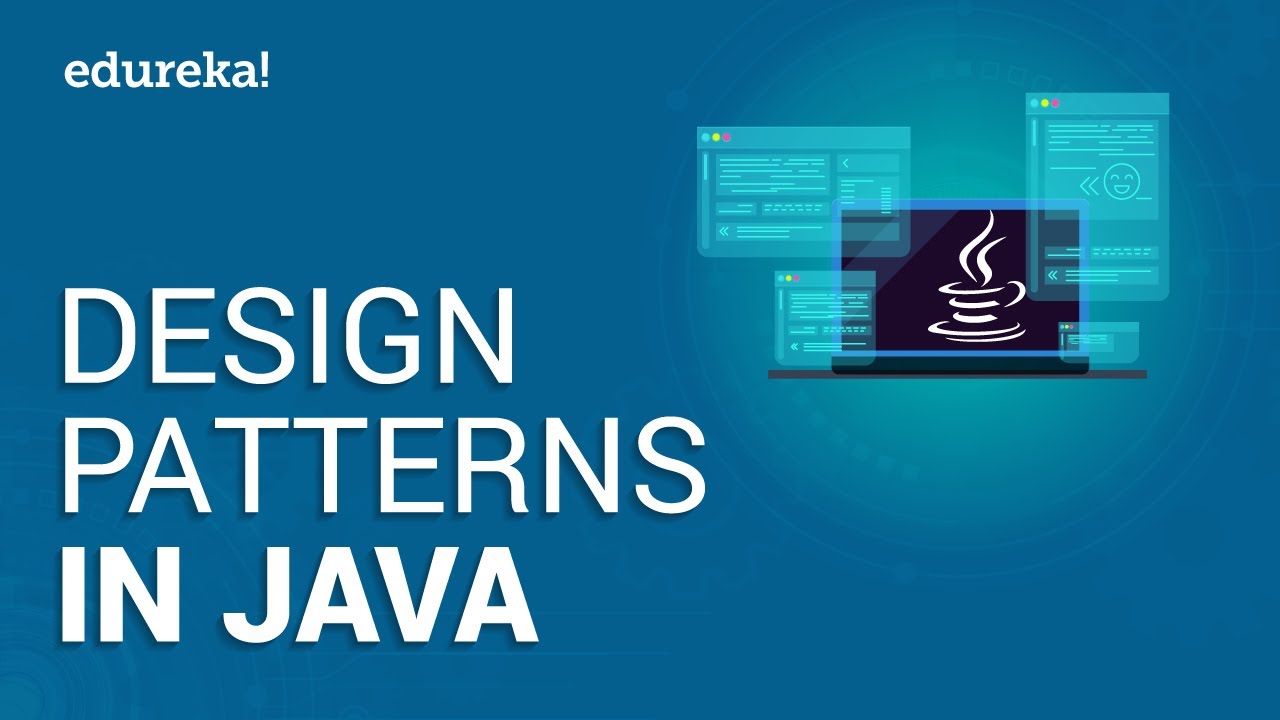
At that time, we only need to create additional subclasses that extend BankAccount and add them to the factory method. By using the Visitor pattern in this scenario, you can add new types of tasks or visitors to the zoo without changing the existing animal classes. This makes it easier to manage and extend the zoo’s functionality over time. The Singleton Pattern restricts the instantiation of a Class and ensures that only one instance of the class exists in the Java Virtual Machine (JVM). The implementation of the Singleton Pattern has always been a controversial topic among developers.
Over time, the most advantageous solutions were obtained through trial and error, along with a great deal of experimentation involving many ideas and approaches. These solutions have now been codified into a set of recognized design patterns. Professional software developers are expected to know at least some popular solutions to typical coding problems.
No comments:
Post a Comment